Laravel 10: new major release
As some of you already know, since it has been announced last year, the Laravel team will no longer work with LTS (long term support) releases but with annual major version bumps. After a year of active development on Laravel 10 and active support on Laravel 9, the release is finally here. Surely a lot of structural changes happened in this release. To give you a little hint, on Twitter and Reddit many enthusiasts have already named L10: The king of types.
Artisan becomes more interactive
From Laravel 10 on, Artisan becomes more interactive.
For example: you ask to create a model, but you forget to pass the name:
1
php artisan make:model
Instead of giving an error message, Artisan will simply ask you the name of the model, as well as whether you want to create a migration, factory, etc. Very handy!
1
2
3
4
5
6
7
8
9
10
11
12
13
14
php artisan make:model
What should the model be named?
❯ Post
Would you like any of the following? [none]
none ......................................................................................... 0
all .......................................................................................... 1
factory ...................................................................................... 2
form requests ................................................................................ 3
migration .................................................................................... 4
policy ....................................................................................... 5
resource controller .......................................................................... 6
seed ......................................................................................... 7
Is your application up to date?
An up-to-date application not only gives you the latest features and improvements, but also maximizes security and performance.
The Laravel 10 skeleton uses native types instead of docblocks
Also from Laravel 10 on, the skeleton will use native types instead of docblocks.
The team has also added generic type annotations, which will dramatically improve auto-completion when coding as well (if your code editor supports generic annotations).
See the PR on GitHub: [10.x] PHP uses Native Type Declarations 🐘
For example, in the Laravel skeleton, the schedule() method in app/Console/Kernel.php will look like this 🤩
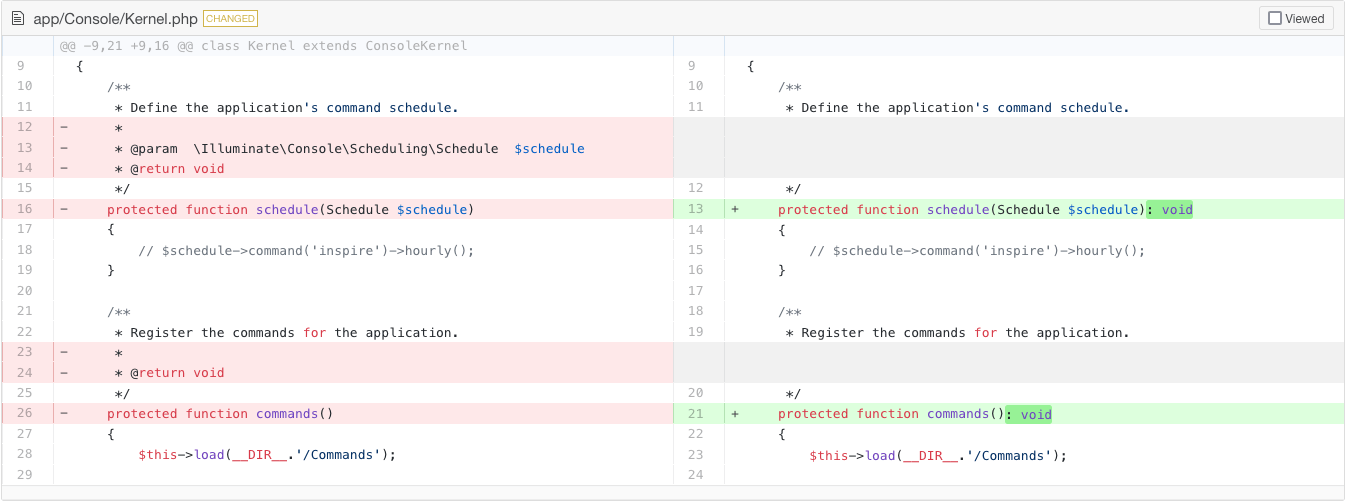
Official packages will also use native types
Actually, you don't have to wait until Laravel 10 to enjoy native type hints in official Laravel packages. The Laravel core dev team was already integrating this throughout the year, which has certainly already paid off:
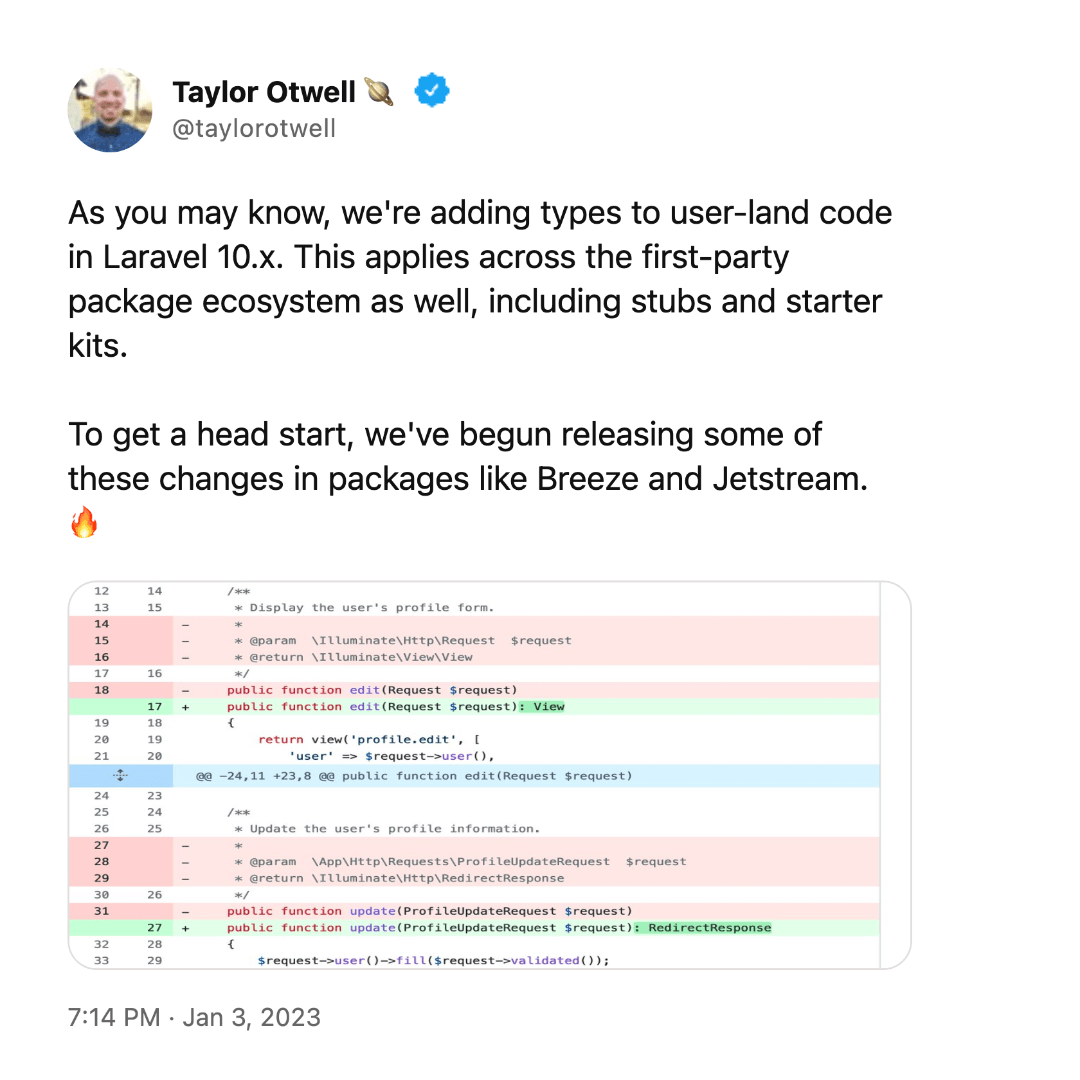
No more support for PHP 8.0
If Laravel 10 drops support for PHP 8.0 and requires at least 8.1, that means two things if you want to upgrade:
Either switch to PHP 8.1
Or PHP 8.2
Why are they dropping PHP 8.0 support already? PHP 8.0 has not been in Active support since Nov 26, 2022, so it only falls under Security fixes now. Since Laravel is also no longer working with LTS versions, the vision from Laravel is that they go with the technology currently in the community as best they can. Staying stuck on PHP 8.0 would therefore not fit with their new vision.
You can find the pull request on GitHub: [10.x] Drop PHP 8.0
Doctrine/dbal is no longer needed to change columns in migrations
In an effort to eliminate the need for doctrine/dbal package when using change() to modify columns, a new feature is coming to Laravel 10. This feature will allow developers to use the change() method and modify columns on MySQL, PostgreSQL, and SQL Server without the need for any additional packages. This is a significant and risky change, but we do believe it is worth the effort.
To better understand the new feature, see this example:
1
2
3
$table->integer('user_balance')->unsigned()->default(0);
// `user_balance` is an integer, unsigned, with a default value of '0'.
Now we assume we have a column for user_balance and we want to change its type. Since Laravel 10, we can do this easily:
1
2
3
$table->bigInteger('user_balance')->change();
// This changes `user_balance` to a bigInteger instead of an integer
The above code successfully changes the type of the column, but also drops the attributes UNSIGNED and DEFAULT. Therefore, it is important to remember to add all attributes when changing the type of a column:
1
$table->bigInteger('user_balance')->unsigned()->default(0)->change();
In case you have multiple database connections and have doctrine/dbal already installed, it is recommended that you call the Schema::useNativeSchemaOperationsIfPossible() method within the boot method in AppProvidersAppServiceProvider to be able to use native schema operations and to use native operations before relying on the package (SQLite, for example, does not yet support this):
1
2
3
4
5
6
7
8
9
use IlluminateSupportFacadesSchema;
class AppServiceProvider extends ServiceProvider
{
public function boot()
{
Schema::useNativeSchemaOperationsIfPossible();
}
}
Retrieve column types
Another notable feature of Laravel 10 is the ability to use the Schema::getColumnType method without depending on the doctrine/dbal package. Currently, we use Schema::getColumnType with doctrine/dbal to get the column type. Doctrine/dbal maps each native column type to its doctrine/dbal type equivalent, and it does not support many of the column types used by Laravel in different databases.
In Laravel 10, by contrast, the new method Schema::getColumnType returns the actual column type instead of the doctrine/dbal equivalent. This also allows you to write integration tests for the new native column change function. You can use this function to get the data type name or the entire type definition of the specified column:
Deprecations of Laravel 9
Methods that were marked as deprecated in Laravel 9 will be removed in Laravel 10. The upgrade guide that you can find back in the documentation contains a list of the deprecated methods and the potential consequences.
Here are some deprecations we found:
- Removal of several deprecations PR #41136
- Removing deprecated date properties PR #41136
- Removing the handleDeprecation method
PR #42590
- Remove the assertTimesSent method
PR #42592
- Removing the ScheduleListCommand's $defaulName property
Diff 419471e
- Removing the deprecated method Route::home
PR #42614
- Removal of deprecated dispatchNow functionality
PR 42591
Thinking about digitalization yourself?
At Codana you can ask all your questions and together we will work out a plan with a sustainable outlook for the future!
Laravel Pennant - Feature flag system
Laravel Pennant is a package published by the Laravel team along with the release of Laravel 10. Pennant allows working with feature flags without requiring community packages.
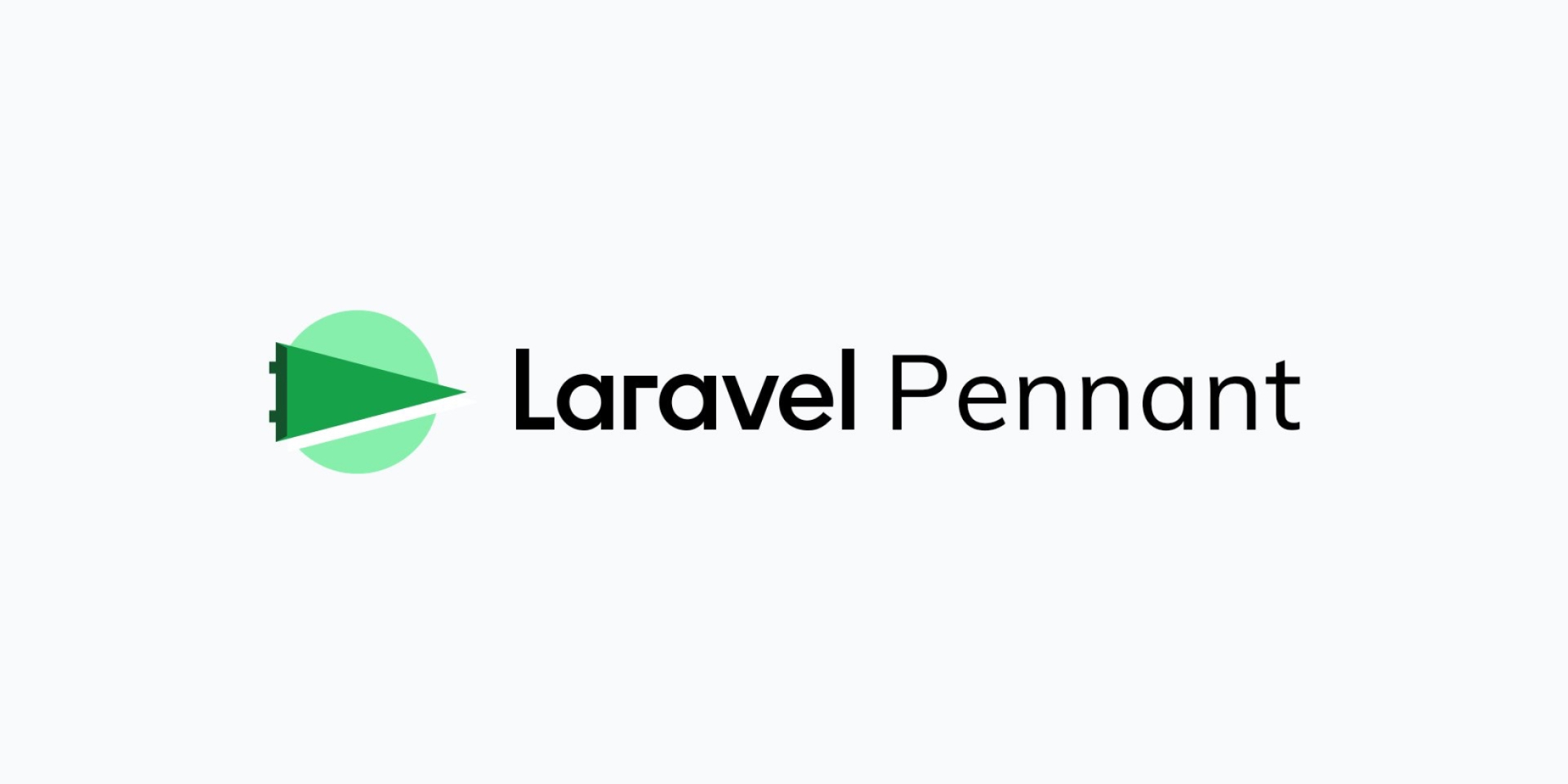
You can install Laravel Pennant very easily with composer:
1
composer require laravel/pennant
To give a brief example of how feature flags work, you can deploy a functionality only to a select group of users in your production environment. This is great for A/B testing, for example.
1
2
3
4
5
6
use Laravel\Pennant\Feature;
use Illuminate\Support\Lottery;
Feature::define('new-coupon-order-flow', function () {
return Lottery::odds(1, 10);
});
Verify that the user has access to the function:
1
2
3
if (Feature::active('new-coupon-order-flow')) {
//
}
There is even a Blade directive:
1
2
3
@feature('new-coupon-order-flow')
…
@endfeature
To learn more about Laravel Pennant, take a look at the official documentation.